Week 1 - First Engineering & Design Lesson
Week 1 - 19 October 2016
It was our first lesson after the holidays and we were briefed about the module Engineering & Design. After that, we had a class activity to carry out which involved Arduino. It had been along time since we touched and used an Arduino as such we took quite sometime to do the activity.
Nontheless, we managed to complete the activity from the help of our classmates, teachers and the internet.
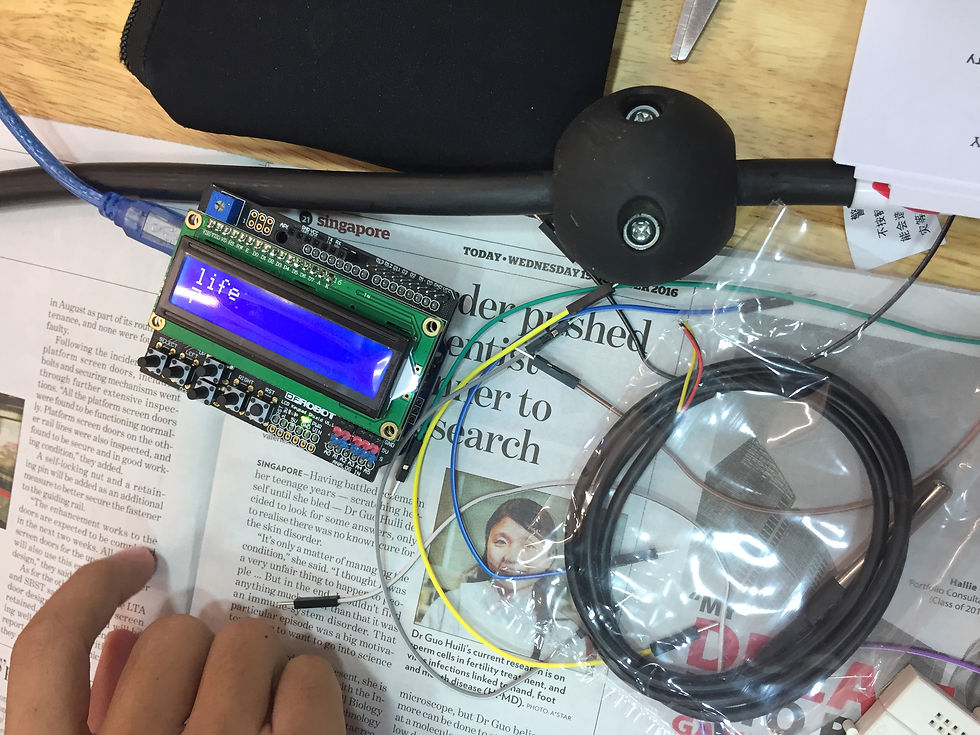
Our first activity was Data Acquisition.
Data Acquisition is the process of sampling signals, that measures real world parameters, in digital numeric values which could be processed if required.
TASK 1 : Connect LCD to Arduino
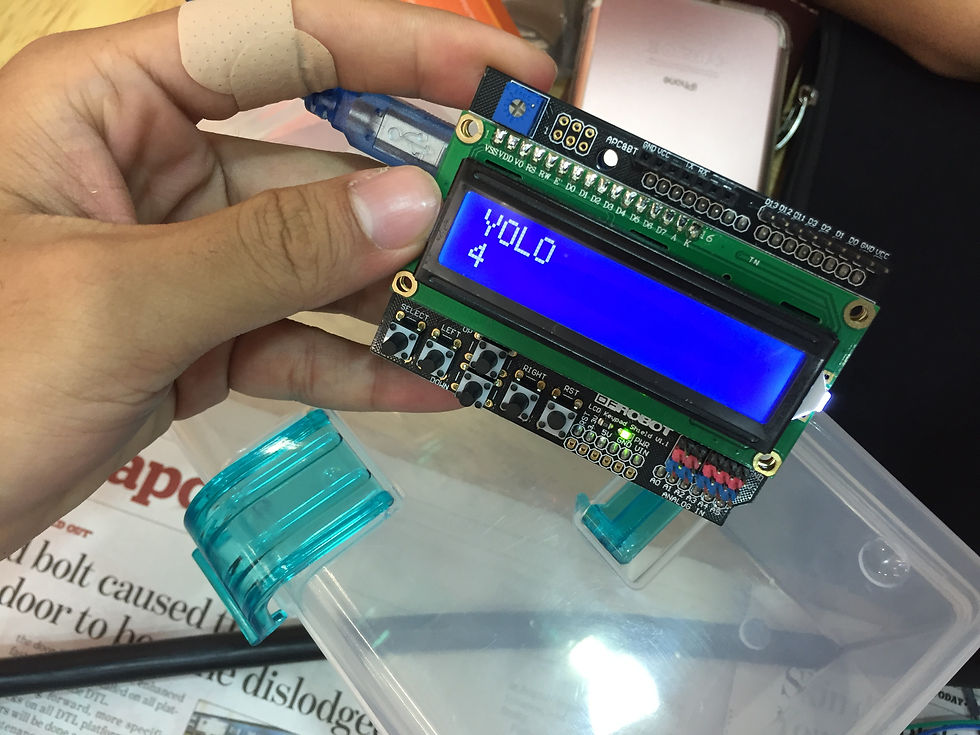
Pins used : 8, 9, 4, 5, 6, 7, VCC and Ground Arduino Code to test that the LCD is properly connected #include <LiquidCrystal.h> //initialize the library with the numbers of the interface pins LiquidCrystal lcd(8, 9, 4, 5, 6, 7);
void setup( ) { // set up the LCD's number of columns and rows: lcd.begin(16, 2);
// Print a message to the LCD.
lcd.print("YOLO");
}
void loop( ) {
// set the cursor to column 0, line
// (note: line 1 is the second row, since counting begins with 0):
lcd.setCursor(0, 1);
// print the number of seconds since reset: lcd.print(millis( ) / 1000);
}
TASK 2 : Sensor Interfacing
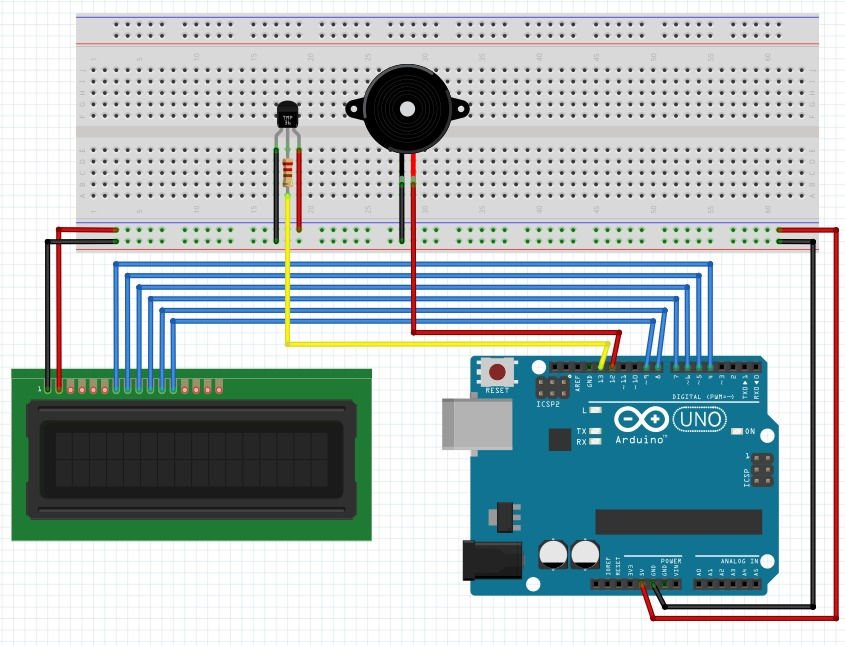
Circuit Layout for connecting Temperature Sensor, Resistor, LCD Display Screen and Arduino
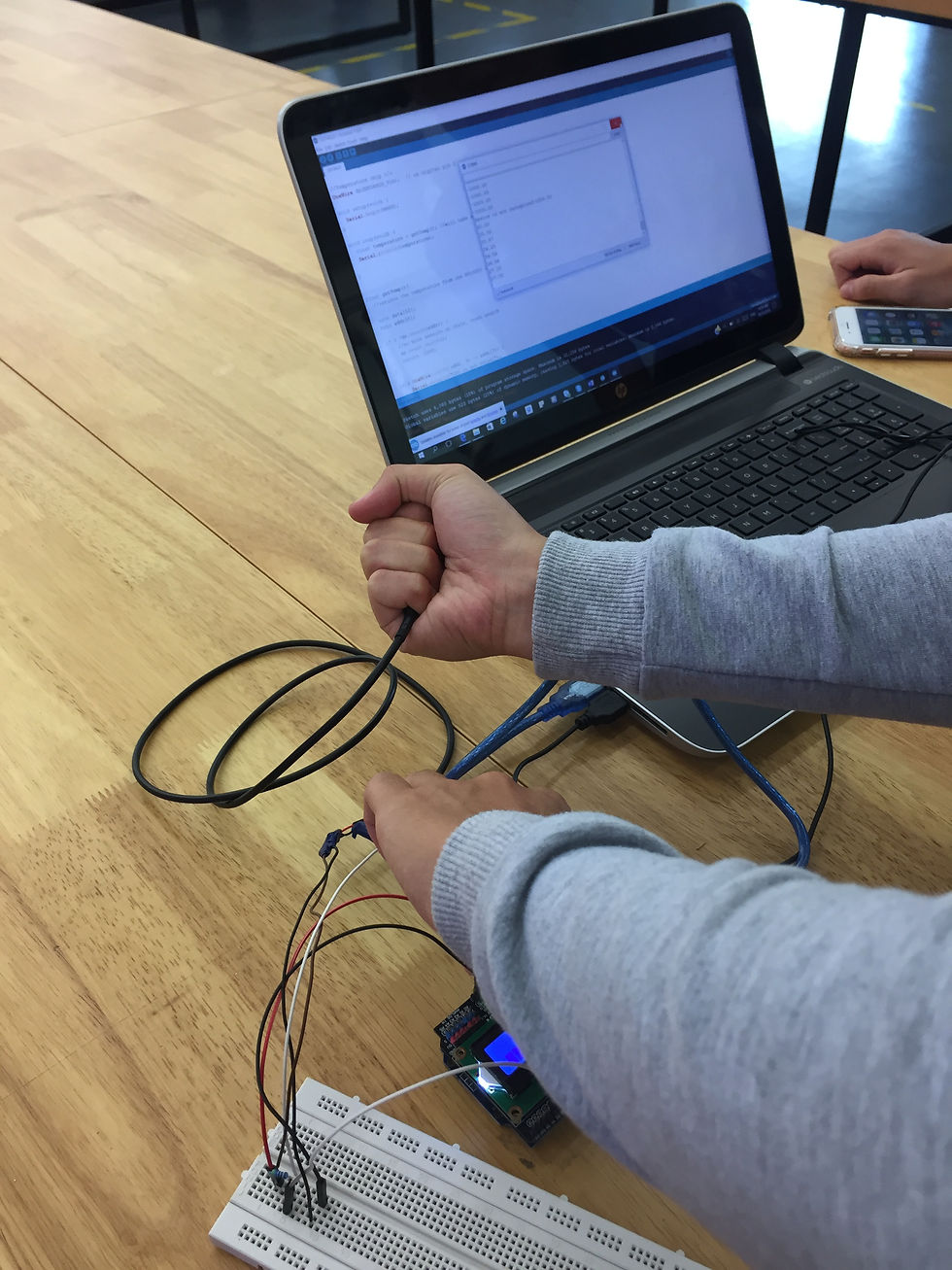

Temperature Measurements from the sensor
Sensor D - Temperature
Digital or Analog : Digital
Arduino Interface : One Wire
Operating Temperature : -55 to 125 Degrees Celsius
Range : 180 Degrees Celsius
Pins Used : D2, VCC, Ground
Temperature Sensor requires no calibration.
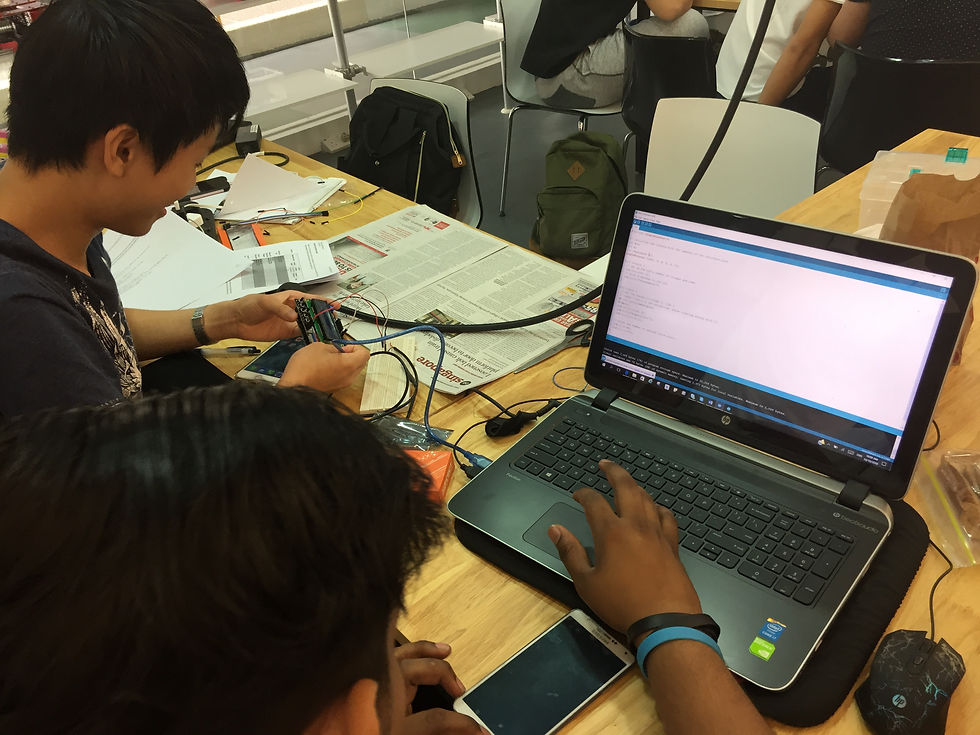

TASK 3 : Alarm System activates when temperature is above 32 Degree Celsius
Arduino Code for Alarm system when temperature is above 32 Degree Clesius
int DS18S20_Pin = 2; //DS18S20 Signal pin on digital 2 int Buzz = 13; int threshold = 32 ;
//Temperature chip i/o OneWire ds(DS18S20_Pin); // on digital pin 2 LiquidCrystal lcd(8, 9, 4, 5, 6, 7); void setup(void) { Serial.begin(9600); // set up the LCD's number of columns and rows: lcd.begin(16, 2); // Print a message to the LCD. lcd.print("Temperature:"); pinMode(Buzz, OUTPUT); }
void loop(void) { float temperature = getTemp() ;//will take about 750ms to run int Buz = temperature; // set the cursor to column 0, line 1 // (note: line 1 is the second row, since counting begins with 0): lcd.setCursor(0, 1); lcd.print("Celcius"); // print the number of seconds since reset: lcd.setCursor(9, 1); lcd.print(temperature); if (Buz >= threshold) { digitalWrite(Buzz, HIGH); } else { digitalWrite(Buzz, LOW); } Serial.println(Buz); Serial.println(threshold);
Serial.println(temperature); }
float getTemp(){ //returns the temperature from one DS18S20 in DEG Celsius
byte data[12]; byte addr[8];
if ( !ds.search(addr)) { //no more sensors on chain, reset search ds.reset_search(); return -1000; }
if ( OneWire::crc8( addr, 7) != addr[7]) { Serial.println("CRC is not valid!"); return -1000; }
if ( addr[0] != 0x10 && addr[0] != 0x28) { Serial.print("Device is not recognized"); return -1000; }
ds.reset(); ds.select(addr); ds.write(0x44,1); // start conversion, with parasite power on at the end delay(750); // Wait for temperature conversion to complete
byte present = ds.reset(); ds.select(addr); ds.write(0xBE); // Read Scratchpad
for (int i = 0; i < 9; i++) { // we need 9 bytes data[i] = ds.read(); } ds.reset_search(); byte MSB = data[1]; byte LSB = data[0];
float tempRead = ((MSB << 8) | LSB); //using two's compliment float TemperatureSum = tempRead / 16; return TemperatureSum; }
TASK 4 : Knowledge Sharing
We walked over to group 6 to know more about their sensor which was a conductivity sensor
Sensor C - Conductivity Sensor
Digital or Analog : Analog
Arduino Interface : UART Operating Temperature : 1 to 100 Degree Celsius
Range : 5 to 200,000 ms/cm
Pins Used : D4
1. Is there any difference in the type of output signals of the sensor? Does it affect the selection of the port to which it is interfaced with the Arduino?
Yes. A temperature sensor produces a digital output while a conductivity sensor produces an analog output signal. Yes, it does affect the port selection.
2. How does the calibration procedure for your sensor differ from that of the other type of sensor?
The temperature sensor does not require any calibration.
3. Find our why is it necessary to monitor the parameter of the other group's sensor in lakes or in a process industry?
Conductivity measures the water's ability to conduct electricity.
It is necessary as aquatic animals and plants are adapted to survive in a certain range of salinity/conductivity.
If the conductivity is not in the appropriate range, they will be negatively affected and may die.
Edited by Fang Yi